MyBatis-Plus 快速入門
1.簡介
MyBatis-Plus (簡稱 MP)是一個 MyBatis 的增強工具,在 MyBatis 的基礎上只做增強不做改變,為簡化開發、提高效率而生。
1.1.特性
- 無侵入:只做增強不做改變,引入它不會對現有工程產生影響,如絲般順滑
- 損耗小:啟動即會自動注入基本 CURD,性能基本無損耗,直接面向對象操作
- 強大的 CRUD 操作:內置通用 Mapper、通用 Service,僅僅通過少量配置即可實現單表大部分 CRUD 操作,更有強大的條件構造器,滿足各類使用需求
- 支持 Lambda 形式調用:通過 Lambda 表達式,方便的編寫各類查詢條件,無需再擔心字段寫錯
- 支持主鍵自動生成:支持多達 4 種主鍵策略(內含分佈式唯一 ID 生成器 – Sequence),可自由配置,完美解決主鍵問題
- 支持 ActiveRecord 模式:支持 ActiveRecord 形式調用,實體類只需繼承 Model 類即可進行強大的 CRUD 操作
- 支持自定義全局通用操作:支持全局通用方法注入( Write once, use anywhere )
- 內置代碼生成器:採用代碼或者 Maven 插件可快速生成 Mapper 、 Model 、 Service 、 Controller 層代碼,支持模板引擎,更有超多自定義配置等您來使用
- 內置分頁插件:基於 MyBatis 物理分頁,開發者無需關心具體操作,配置好插件之後,寫分頁等同於普通 List 查詢
- 分頁插件支持多種數據庫:支持 MySQL、MariaDB、Oracle、DB2、H2、HSQL、SQLite、Postgre、SQLServer 等多種數據庫
- 內置性能分析插件:可輸出 SQL 語句以及其執行時間,建議開發測試時啟用該功能,能快速揪出慢查詢
- 內置全局攔截插件:提供全表 delete 、 update 操作智能分析阻斷,也可自定義攔截規則,預防誤操作
1.2.支持數據庫
任何能使用
mybatis
進行 CRUD, 並且支持標準 SQL 的數據庫,具體支持情況如下,如果不在下列表查看分頁部分教程 PR 您的支持。
- mysql,oracle,db2,h2,hsql,sqlite,postgresql,sqlserver,Phoenix,Gauss ,clickhouse,Sybase,OceanBase,Firebird,cubrid,goldilocks,csiidb
- 達夢數據庫,虛谷數據庫,人大金倉數據庫,南大通用(華庫)數據庫,南大通用數據庫,神通數據庫,瀚高數據庫
1.3.框架結構
2.快速上手
2.1.pom.xml導入MyBatis Plus依賴
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>Latest Version</version>
</dependency>
2.2.在application.yml中配置數據源
# 配置數據源
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/wyl? useSSL=false&useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8
username: root
password: 123456
# 配置日誌,輸出更詳細日誌信息
mybatis-plus:
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
2.3.根據數據庫表創建實體類
@Data
@AllArgsConstructor
@NoArgsConstructor
public class User {
private Long id;
private String name;
private Integer age;
private String email;
}
2.4.創建Mapper接口
//在對應的mapper上面繼承基本的接口BaseMapper
@ResponseBody //代表持久層
public interface UserMapper extends BaseMapper<User> {
//所有的CRUD操作都已經編寫完成了
//你不需要像以前的配置一大堆文件了
}
2.5.測試
啟動類需要加 @MapperScan(“mapper所在的包”),否則無法加載mapper bean
@SpringBootTest
class MybatisPlusApplicationTests {
//繼承了BaseMapper,所有的方法都來自父類
//我們也可以編寫自己的擴展方法
@Autowired
private UserMapper userMapper;
@Test
void contextLoads() {
//參數是一個Wrapper,條件構造器,這裡我們先不用 null
//查詢全部用戶
List<User> users = userMapper.selectList(null);
users.forEach(System.out::println);
}
}
那麼我的sql 誰寫的,方法又去哪了,其實都是mybatis plus。
3.配置日誌
我們所有的sql現在是不可見的,我們希望知道他是怎麼執行的,所以我們必須要看日誌!
# 配置日誌
mybatis-plus.configuration.log-impl=org.apache.ibatis.logging.stdout.StdOutImpl
4.CRUD
4.1.Service CRUD 接口
4.1.1.Save
// 插入一條記錄(選擇字段,策略插入)
boolean save(T entity);
// 插入(批量)
boolean saveBatch(Collection<T> entityList);
// 插入(批量)
boolean saveBatch(Collection<T> entityList, int batchSize);
4.1.2.SaveOrUpdate
// TableId 註解存在更新記錄,否插入一條記錄
boolean saveOrUpdate(T entity);
// 根據updateWrapper嘗試更新,否繼續執行saveOrUpdate(T)方法
boolean saveOrUpdate(T entity, Wrapper<T> updateWrapper);
// 批量修改插入
boolean saveOrUpdateBatch(Collection<T> entityList);
// 批量修改插入
boolean saveOrUpdateBatch(Collection<T> entityList, int batchSize);
4.1.3.Remove
// 根據 entity 條件,刪除記錄
boolean remove(Wrapper<T> queryWrapper);
// 根據 ID 刪除
boolean removeById(Serializable id);
// 根據 columnMap 條件,刪除記錄
boolean removeByMap(Map<String, Object> columnMap);
// 刪除(根據ID 批量刪除)
boolean removeByIds(Collection<? extends Serializable> idList);
4.1.4.Update
// 根據 UpdateWrapper 條件,更新記錄 需要設置sqlset
boolean update(Wrapper<T> updateWrapper);
// 根據 whereWrapper 條件,更新記錄
boolean update(T updateEntity, Wrapper<T> whereWrapper);
// 根據 ID 選擇修改
boolean updateById(T entity);
// 根據ID 批量更新
boolean updateBatchById(Collection<T> entityList);
// 根據ID 批量更新
boolean updateBatchById(Collection<T> entityList, int batchSize);
4.1.5.Get
// 根據 ID 查詢
T getById(Serializable id);
// 根據 Wrapper,查詢一條記錄。結果集,如果是多個會拋出異常,隨機取一條加上限制條件 wrapper.last("LIMIT 1")
T getOne(Wrapper<T> queryWrapper);
// 根據 Wrapper,查詢一條記錄
T getOne(Wrapper<T> queryWrapper, boolean throwEx);
// 根據 Wrapper,查詢一條記錄
Map<String, Object> getMap(Wrapper<T> queryWrapper);
// 根據 Wrapper,查詢一條記錄
<V> V getObj(Wrapper<T> queryWrapper, Function<? super Object, V> mapper);
4.1.6.List
// 查詢所有
List<T> list();
// 查詢列表
List<T> list(Wrapper<T> queryWrapper);
// 查詢(根據ID 批量查詢)
Collection<T> listByIds(Collection<? extends Serializable> idList);
// 查詢(根據 columnMap 條件)
Collection<T> listByMap(Map<String, Object> columnMap);
// 查詢所有列表
List<Map<String, Object>> listMaps();
// 查詢列表
List<Map<String, Object>> listMaps(Wrapper<T> queryWrapper);
// 查詢全部記錄
List<Object> listObjs();
// 查詢全部記錄
<V> List<V> listObjs(Function<? super Object, V> mapper);
// 根據 Wrapper 條件,查詢全部記錄
List<Object> listObjs(Wrapper<T> queryWrapper);
// 根據 Wrapper 條件,查詢全部記錄
<V> List<V> listObjs(Wrapper<T> queryWrapper, Function<? super Object, V> mapper);
4.1.7.Page
// 無條件分頁查詢
IPage<T> page(IPage<T> page);
// 條件分頁查詢
IPage<T> page(IPage<T> page, Wrapper<T> queryWrapper);
// 無條件分頁查詢
IPage<Map<String, Object>> pageMaps(IPage<T> page);
// 條件分頁查詢
IPage<Map<String, Object>> pageMaps(IPage<T> page, Wrapper<T> queryWrapper);
4.1.8.Count
// 查詢總記錄數
int count();
// 根據 Wrapper 條件,查詢總記錄數
int count(Wrapper<T> queryWrapper);
4.1.9.Chain
query
// 鏈式查詢 普通
QueryChainWrapper<T> query();
// 鏈式查詢 lambda 式。注意:不支持 Kotlin
LambdaQueryChainWrapper<T> lambdaQuery();
// 示例:
query().eq("column", value).one();
lambdaQuery().eq(Entity::getId, value).list();
update
// 鏈式更改 普通
UpdateChainWrapper<T> update();
// 鏈式更改 lambda 式。注意:不支持 Kotlin
LambdaUpdateChainWrapper<T> lambdaUpdate();
// 示例:
update().eq("column", value).remove();
lambdaUpdate().eq(Entity::getId, value).update(entity);
4.2.Mapper CRUD 接口
4.2.1.Insert
// 插入一條記錄
int insert(T entity);
4.2.2.Delete
// 根據 entity 條件,刪除記錄
int delete(@Param(Constants.WRAPPER) Wrapper<T> wrapper);
// 刪除(根據ID 批量刪除)
int deleteBatchIds(@Param(Constants.COLLECTION) Collection<? extends Serializable> idList);
// 根據 ID 刪除
int deleteById(Serializable id);
// 根據 columnMap 條件,刪除記錄
int deleteByMap(@Param(Constants.COLUMN_MAP) Map<String, Object> columnMap);
4.2.3.Update
// 根據 whereWrapper 條件,更新記錄
int update(@Param(Constants.ENTITY) T updateEntity, @Param(Constants.WRAPPER) Wrapper<T> whereWrapper);
// 根據 ID 修改
int updateById(@Param(Constants.ENTITY) T entity);
4.2.4.Select
// 根據 ID 查詢
T selectById(Serializable id);
// 根據 entity 條件,查詢一條記錄
T selectOne(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
// 查詢(根據ID 批量查詢)
List<T> selectBatchIds(@Param(Constants.COLLECTION) Collection<? extends Serializable> idList);
// 根據 entity 條件,查詢全部記錄
List<T> selectList(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
// 查詢(根據 columnMap 條件)
List<T> selectByMap(@Param(Constants.COLUMN_MAP) Map<String, Object> columnMap);
// 根據 Wrapper 條件,查詢全部記錄
List<Map<String, Object>> selectMaps(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
// 根據 Wrapper 條件,查詢全部記錄。注意: 只返回第一個字段的值
List<Object> selectObjs(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
// 根據 entity 條件,查詢全部記錄(並翻頁)
IPage<T> selectPage(IPage<T> page, @Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
// 根據 Wrapper 條件,查詢全部記錄(並翻頁)
IPage<Map<String, Object>> selectMapsPage(IPage<T> page, @Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
// 根據 Wrapper 條件,查詢總記錄數
Integer selectCount(@Param(Constants.WRAPPER) Wrapper<T> queryWrapper);
案例
List<User> userList1 = user.selectList(
new EntityWrapper<User>().eq("name", "王延領")
);
分頁
// 分頁查詢 10 條姓名為『wyl』的用戶記錄
List<User> userList = user.selectPage(
new Page<User>(1, 10),
new EntityWrapper<User>().eq("name", "wyl")
).getRecords();
結合
// 分頁查詢 10 條姓名為『wyl』、性別為男,且年齡在18至50之間的用戶記錄
List<User> userList = userMapper.selectPage(
new Page<User>(1, 10),
new EntityWrapper<User>().eq("name", "wyl")
.eq("sex", 0)
.between("age", "18", "50")
);
4.3.mapper 層 選裝件
AlwaysUpdateSomeColumnById
int alwaysUpdateSomeColumnById(T entity);
insertBatchSomeColumn
int insertBatchSomeColumn(List<T> entityList);
logicDeleteByIdWithFill
int logicDeleteByIdWithFill(T entity);
4.4.條件構造器
十分重要:Wappper
我們寫一些複雜的SQL就可以使用他來替代!
1、測試一,記住查看輸出的SQL進行分析
@Test
void contextLoads() {
//查詢name不為空的用戶,並且郵箱不為空的用戶,年齡大於12
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.isNotNull("name")
.isNotNull("email")
.ge("age", 12);
userMapper.selectList(wrapper).forEach(System.out::println); //和我們剛剛學習的map對比一下
}
2、測試二,記住查看輸出的SQL進行分析
@Test
void test2(){
//查詢名字Chanv
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.eq("name", "Chanv");
User user = userMapper.selectOne(wrapper);
System.out.println(user);
}
3、測試三
@Test
void test3(){
//查詢年齡在19到30歲之間的用戶
QueryWrapper<User> wrapper = new QueryWrapper<>();
wrapper.between("age", 19, 30); //區間
Integer count = userMapper.selectCount(wrapper);
System.out.println(count);
}
4、測試四,記住查看輸出的SQL進行分析
//模糊查詢
@Test
void test4(){
//查詢年齡在19到30歲之間的用戶
QueryWrapper<User> wrapper = new QueryWrapper<>();
//左和右
wrapper.notLike("name", "b")
.likeRight("email", "t");
List<Map<String, Object>> maps = userMapper.selectMaps(wrapper);
maps.forEach(System.out::println);
}
5、測試五
@Test
void test5(){
QueryWrapper<User> wrapper = new QueryWrapper<>();
//id 在子查詢中查出來
wrapper.inSql("id", "select id from user where id < 3");
List<Object> objects = userMapper.selectObjs(wrapper);
objects.forEach(System.out::println);
}
6、測試六
@Test
void test6(){
QueryWrapper<User> wrapper = new QueryWrapper<>();
//通過id進行排序
wrapper.orderByDesc("id");
List<User> users = userMapper.selectList(wrapper);
users.forEach(System.out::println);
}
5.代碼生成器
dao、pojo、service、controller都給我自己去編寫完成!
AutoGenerator 是 MyBatis-Plus 的代碼生成器,通過 AutoGenerator 可以快速生成 Entity、Mapper、Mapper XML、Service、Controller 等各個模塊的代碼,極大的提升了開發效率。
5.1.導入依賴
<!-- 代碼生成器依賴 -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.3.1.tmp</version>
</dependency>
<!-- 生成器需要根據模板生成各種組件,所以模板也需要導入 -->
<!-- velocity是默認的模板,除了它以外常用的還有:Freemarker、Beetl -->
<dependency>
<groupId>org.apache.velocity</groupId>
<artifactId>velocity</artifactId>
<version>1.7</version>
</dependency>
5.2、啟動類,任意一個main、@Test方法都行
package com.wyl.mybatisplus;
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.config.DataSourceConfig;
import com.baomidou.mybatisplus.generator.config.GlobalConfig;
import com.baomidou.mybatisplus.generator.config.PackageConfig;
import com.baomidou.mybatisplus.generator.config.StrategyConfig;
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
public class Code {
public static void main(String[] args) {
//需要構建一個 代碼自動生成器 對象
// 代碼生成器
AutoGenerator mpg = new AutoGenerator();
//配置策略
//1、全局配置
GlobalConfig gc = new GlobalConfig();
String projectPath = System.getProperty("user.dir");
gc.setOutputDir(projectPath + "/src/main/java");
gc.setAuthor("ChanV");
gc.setOpen(false);
gc.setFileOverride(false); //是否覆蓋
gc.setServiceName("%sService"); //去Service的I前綴
gc.setIdType(IdType.ID_WORKER);
gc.setDateType(DateType.ONLY_DATE);
gc.setSwagger2(true);
mpg.setGlobalConfig(gc);
//2、設置數據源
DataSourceConfig dsc = new DataSourceConfig();
dsc.setUrl("jdbc:mysql://localhost:3306/mybatis-plus?useSSL=false&useUnicode=true&characterEncoding=utf-8&serverTimezone=GMT%2B8");
dsc.setDriverName("com.mysql.cj.jdbc.Driver");
dsc.setUsername("root");
dsc.setPassword("root");
dsc.setDbType(DbType.MYSQL);
mpg.setDataSource(dsc);
//3、包的配置
PackageConfig pc = new PackageConfig();
pc.setModuleName("blog");
pc.setParent("com.chanv");
pc.setEntity("pojo");
pc.setMapper("mapper");
pc.setService("service");
pc.setController("controller");
mpg.setPackageInfo(pc);
//4、策略配置
StrategyConfig strategy = new StrategyConfig();
strategy.setInclude("user"); //設置要映射的表名
strategy.setNaming(NamingStrategy.underline_to_camel);
strategy.setColumnNaming(NamingStrategy.underline_to_camel);
strategy.setEntityLombokModel(true); //自動lombok
strategy.setLogicDeleteFieldName("deleted");
//自動填充配置
TableFill createTime = new TableFill("create_time", FieldFill.INSERT);
TableFill updateTime = new TableFill("update_time", FieldFill.UPDATE);
ArrayList<TableFill> tableFills = new ArrayList<>();
tableFills.add(createTime);
tableFills.add(updateTime);
strategy.setTableFillList(tableFills);
//樂觀鎖
strategy.setVersionFieldName("version");
strategy.setRestControllerStyle(true);
strategy.setControllerMappingHyphenStyle(true); //localhost:8080/hello_id_2
mpg.setStrategy(strategy);
mpg.execute(); //執行代碼構造器
}
}
以上兩步即可完成生成代碼功能!
啟動類上掃描
@SpringBootApplication // 啟動類
@MapperScan(value = {"com.wyl.mybatisplus.generator.mapper"}) // 掃描mapper
public class MybatisplusApplication {
public static void main(String[] args) {
SpringApplication.run(MybatisplusApplication.class, args);
}
}
測試類上掃描
@SpringBootTest
@MapperScan(value = {"com.wyl.mybatisplus.generator.mapper"}) // @MapperScan("mapper的包位置")
class UserServiceTest {
@Autowired
private UserMapper mapper;
@Test
public void test(){
mapper.selectList(null).forEach(System.out::println);
}
}
5.3.測試MVC
5.3.1.後端:controller.java
package com.wyl.mybatisplus.generator.controller;
import com.wyl.mybatisplus.generator.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.stereotype.Controller;
import org.springframework.web.servlet.ModelAndView;
@Controller
@RequestMapping("/generator/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/success")
public ModelAndView index(){
ModelAndView mav = new ModelAndView();
mav.setViewName("success");
mav.addObject("list",userService.list());
return mav;
}
}
5.3.2.前端:html
記得放在templates下哦
index.html
<h1>Index Page...</h1>
<a href="/generator/user/success">展示數據</a>
succuss.html
<!DOCTYPE html>
<html lang="en">
<!-- 配置thymeleaf模板標籤庫 -->
<html xmlns:th="//www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Success Page</title>
</head>
<body>
<h1>Success Page...</h1>
<table border="1" cellspacing="0" cellpadding="1">
<tr>
<th>編號</th>
<th>用戶名</th>
<th>年齡</th>
</tr>
<tr th:each="user:${list}">
<td th:text="${user.id}"></td>
<td th:text="${user.userName}"></td>
<td th:text="${user.userAge}"></td>
</tr>
</table>
</body>
</html>
前端用到thymeleaf模板引擎,需要配置application.yml
spring:
# 視圖解析
thymeleaf:
prefix: classpath:/templates/
suffix: .html
6.MybatisX 快速開發插件
MybatisX 是一款基於 IDEA 的快速開發插件,為效率而生。
功能
XML跳轉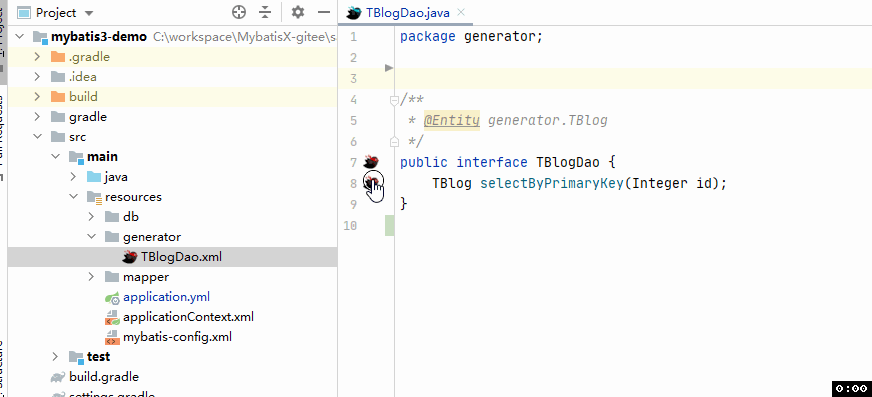
生成代碼(需先在idea配置Database配置數據源)
重置模板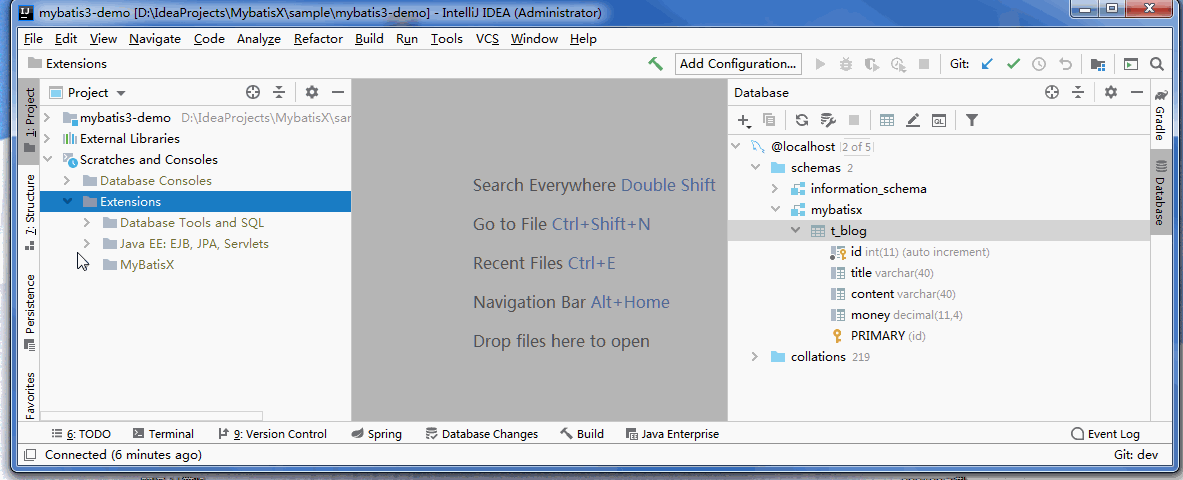
JPA提示
生成新增
生成查詢
生成修改
生成刪除
8.樂觀鎖
在面試過程中,我們經常會被問到樂觀鎖,悲觀鎖!這個其實非常簡單!
原子引用!
樂觀鎖:顧名思義十分樂觀,他總是認為不會出現問題,無論幹什麼不去上鎖!如果出現了問題,再次更新值測試!
悲觀鎖:顧名思義十分悲觀,他總是任務總是出現問題,無論幹什麼都會上鎖!再去操作!
我們這裡主要講解,樂觀鎖機制!
樂觀鎖實現方式:
- 取出記錄,獲取當前version
- 更新時,帶上這個version
- 執行更新時,set version = new version where version = oldversion
- 如果version不對,就更新失敗
樂觀鎖:1、先查詢,獲得版本號 version = 1
-- A
update user set name = "wyl", version = version + 1
where id = 2 and version = 1
-- B 線程搶先完成,這個時候 version = 2,會導致 A 修改失敗!
update user set name = "wjm", version = version + 1
where id = 2 and version = 1
測試一下MP的樂觀鎖插件
1、給數據庫中增加version字段!
//測試樂觀鎖成功!
@Test
public void testOptimisticLocker(){
//1、查詢用戶信息
User user = userMapper.selectById(1330080433207046145L);
//2、修改用戶信息
user.setName("ChanV");
user.setEmail("[email protected]");
//3、執行更新操作
userMapper.updateById(user);
}
//測試樂觀鎖失敗!多線程下
@Test
public void testOptimisticLocker2(){
//線程1
User user = userMapper.selectById(5L);
user.setName("ChanV111");
user.setEmail("[email protected]");
//模擬另一個線程執行了插隊操作
User user2 = userMapper.selectById(5L);
user2.setName("ChanV222");
user2.setEmail("[email protected]");
userMapper.updateById(user2);
//自旋鎖多次嘗試提交
userMapper.updateById(user); //如果沒有樂觀鎖就會覆蓋隊線程的值
}
9.全局策略配置:
通過上面的小案例我們可以發現,實體類需要加@TableName註解指定數據庫表名,通過@TableId註解指定id的增長策略。實體類少倒也無所謂,實體類一多的話也麻煩。所以可以在spring-dao.xml的文件中進行全局策略配置。
<!-- 5、mybatisplus的全局策略配置 -->
<bean id="globalConfiguration" class="com.baomidou.mybatisplus.entity.GlobalConfiguration">
<!-- 2.3版本後,駝峰命名默認值就是true,所以可不配置 -->
<!--<property name="dbColumnUnderline" value="true"/>-->
<!-- 全局主鍵自增策略,0表示auto -->
<property name="idType" value="0"/>
<!-- 全局表前綴配置 -->
<property name="tablePrefix" value="tb_"/>
</bean>
這裡配置了還沒用,還需要在sqlSessionFactory中注入配置才會生效。如下:
<!-- 3、配置mybatisplus的sqlSessionFactory -->
<bean id="sqlSessionFactory" class="com.baomidou.mybatisplus.spring.MybatisSqlSessionFactoryBean">
<property name="dataSource" ref="dataSource" />
<property name="configLocation" value="classpath:mybatis-config.xml"/>
<property name="typeAliasesPackage" value="com.zhu.mybatisplus.entity"/>
<!-- 注入全局配置 -->
<property name="globalConfig" ref="globalConfiguration"/>
</bean>
如此一來,實體類中的@TableName註解和@TableId註解就可以去掉了。