C#開發BIMFACE系列14 服務端API之批量獲取轉換狀態詳情
- 2019 年 10 月 3 日
- 筆記
上一篇《C#開發BIMFACE系列13 服務端API之獲取轉換狀態》中介紹了根據文件ID查詢單個文件的轉換狀態。
本文介紹批量獲取轉換狀態詳情。
請求地址:POST https://api.bimface.com/translateDetails
說明:應用發起轉換以後,可以根據篩選條件,通過該介面批量查詢轉換狀態詳情
參數:
請求 path(示例):https://api.bimface.com/translateDetails
請求 header(示例):“Authorization: Bearer dc671840-bacc-4dc5-a134-97c1918d664b”
請求 body(示例):
{ "appKey" : "appKey", //必填 "endDate" : "string", "fileId" : 0, "fileName" : "fileName", "pageNo" : 0, "pageSize" : 0, "sortType" : "sortType", "sourceId" : "d4649ee227e345c8b7f0022342247dec", "startDate" : "string", "status" : 0, "suffix" : "suffix" }
HTTP響應示例(200):application/octet-stream
{ "code" : "success", "data" : { "list" : [ { "appKey" : "appKey", "cost" : 0, "createTime" : "createTime", "databagId" : "498bc694854244abab728b20620cbaf9", "fileId" : 0, "length" : 0, "name" : "name", "offlineDatabagStatus" : "offlineDatabagStatus", "priority" : 0, "reason" : "reason", "retry" : true, "shareToken" : "shareToken", "shareUrl" : "shareUrl", "sourceId" : "d69620720c63480c9f4808bf442ed96a", "status" : "status", "supportOfflineDatabag" : true, "thumbnail" : [ "string" ], "type" : "type" } ], "page" : { "htmlDisplay" : "string", "nextPage" : 0, "pageNo" : 0, "pageSize" : 0, "prePage" : 0, "startIndex" : 0, "totalCount" : 0, "totalPages" : 0 } }, "message" : "" }
請求體參數說明:
經過測試驗證,其中 appKey 是必填項,其餘參數非必填。
對應封裝的請求實體類為:
1 /// <summary> 2 /// 批量獲取轉換狀態詳情的請求數據 3 /// </summary> 4 [Serializable] 5 public class TranslateQueryRequest 6 { 7 public TranslateQueryRequest() 8 { 9 FileId = null; 10 Suffix = null; 11 FileName = null; 12 SourceId = null; 13 PageNo = null; 14 PageSize = null; 15 Status = null; 16 SortType = null; 17 StartDate = null; 18 EndDate = null; 19 } 20 21 /// <summary> 22 /// 【必填項】應用的 appKey 23 /// </summary> 24 [JsonProperty("appKey")] 25 public string AppKey { get; set; } 26 27 /// <summary> 28 /// 【非必填項】單模型對應的id,例如:1216871503527744 29 /// </summary> 30 [JsonProperty("fileId", NullValueHandling = NullValueHandling.Ignore)] 31 public string FileId { get; set; } 32 33 /// <summary> 34 /// 【非必填項】單模型的文件類型。例如:rvt(或者igms,dwg…) 35 /// </summary> 36 [JsonProperty("suffix", NullValueHandling = NullValueHandling.Ignore)] 37 public string Suffix { get; set; } 38 39 /// <summary> 40 /// 【非必填項】單模型的名稱。例如:translate-test 41 /// </summary> 42 [JsonProperty("fileName", NullValueHandling = NullValueHandling.Ignore)] 43 public string FileName { get; set; } 44 45 /// <summary> 46 /// 【非必填項】模型對應的sourceId。例如:389c28de59ee62e66a7d87ec12692a76 47 /// </summary> 48 [JsonProperty("sourceId", NullValueHandling = NullValueHandling.Ignore)] 49 public string SourceId { get; set; } 50 51 /// <summary> 52 /// 【非必填項】頁碼 53 /// </summary> 54 [JsonProperty("pageNo",NullValueHandling = NullValueHandling.Ignore)] 55 public int? PageNo { get; set; } 56 57 /// <summary> 58 /// 【非必填項】每頁返回數目 59 /// </summary> 60 [JsonProperty("pageSize", NullValueHandling = NullValueHandling.Ignore)] 61 public int? PageSize { get; set; } 62 63 /// <summary> 64 /// 【非必填項】模型狀態碼。1(處理中) 99(成功) -1(失敗) 65 /// </summary> 66 [JsonProperty("status", NullValueHandling = NullValueHandling.Ignore)] 67 public short? Status { get; set; } 68 69 /// <summary> 70 /// 【非必填項】篩選類型 71 /// </summary> 72 [JsonProperty("sortType", NullValueHandling = NullValueHandling.Ignore)] 73 public string SortType { get; set; } 74 75 /// <summary> 76 /// 【非必填項】開始日期。例如:2019-05-01 77 /// </summary> 78 [JsonProperty("startDate", NullValueHandling = NullValueHandling.Ignore)] 79 public string StartDate { get; set; } 80 81 /// <summary> 82 /// 【非必填項】截止日期。例如:2019-05-03 83 /// </summary> 84 [JsonProperty("endDate", NullValueHandling = NullValueHandling.Ignore)] 85 public string EndDate { get; set; } 86 }
C#實現方法:
1 /// <summary> 2 /// 批量獲取轉換狀態詳情 3 /// </summary> 4 /// <param name="accessToken">令牌</param> 5 /// <param name="request">請求體參數對象</param> 6 /// <returns></returns> 7 public virtual FileTranslateDetailsResponse GetFileTranslateDetails(string accessToken, TranslateQueryRequest request) 8 { 9 // POST https://api.bimface.com/translateDetails 10 string url = string.Format(BimfaceConstants.API_HOST + "/translateDetails"); 11 12 BimFaceHttpHeaders headers = new BimFaceHttpHeaders(); 13 headers.AddOAuth2Header(accessToken); 14 15 string data = request.SerializeToJson(); 16 17 try 18 { 19 FileTranslateDetailsResponse response; 20 21 HttpManager httpManager = new HttpManager(headers); 22 HttpResult httpResult = httpManager.Post(url,data); 23 if (httpResult.Status == HttpResult.STATUS_SUCCESS) 24 { 25 response = httpResult.Text.DeserializeJsonToObject<FileTranslateDetailsResponse>(); 26 } 27 else 28 { 29 response = new FileTranslateDetailsResponse 30 { 31 Message = httpResult.RefText 32 }; 33 } 34 35 return response; 36 } 37 catch (Exception ex) 38 { 39 throw new Exception("[批量獲取轉換狀態詳情]發生異常!", ex); 40 } 41 }
其中調用到的 httpManager.Post() 方法,請參考《C# HTTP系列》
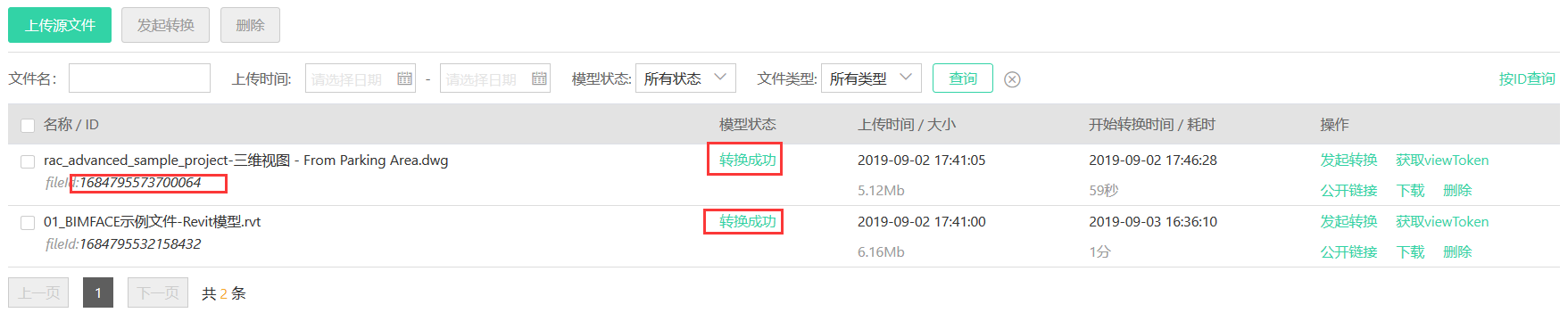
調用上面的GetFileTranslateDetails()方法測試批量查詢轉換狀態:
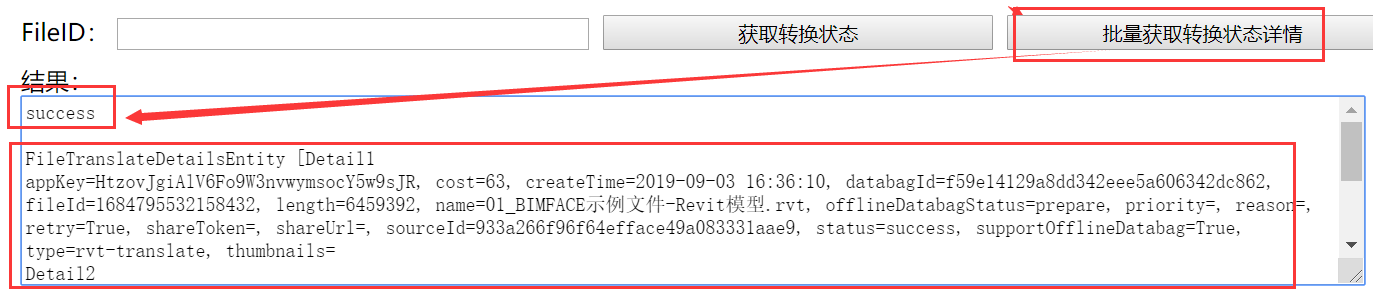
測試程式碼如下:
1 // 批量獲取轉換狀態詳情 2 protected void btnGetFileTranslateDetails_Click(object sender, EventArgs e) 3 { 4 TranslateQueryRequest request = new TranslateQueryRequest 5 { 6 AppKey = _appKey //必填項 7 }; 8 9 FileConvertApi api = new FileConvertApi(); 10 FileTranslateDetailsResponse response = api.GetFileTranslateDetails(txtAccessToken.Text, request); 11 12 txtResult.Text = response.Code.ToString2() 13 + Environment.NewLine 14 + response.Message.ToString2() 15 + Environment.NewLine 16 + response.Data.ToString2(); 17 }
返回的結果實體類如下:
1 /// <summary> 2 /// 批量獲取轉換狀態詳情返回的結果類 3 /// </summary> 4 [Serializable] 5 public class FileTranslateDetailsResponse : GeneralResponse<FileTranslateDetailsEntity> 6 { 7 8 }
1 [Serializable] 2 public class FileTranslateDetailsEntity 3 { 4 [JsonProperty("list")] 5 public Detail[] Details { get; set; } 6 7 [JsonProperty("page")] 8 public Page Page { get; set; } 9 10 /// <summary>返回表示當前對象的字元串。</summary> 11 /// <returns>表示當前對象的字元串。</returns> 12 public override string ToString() 13 { 14 StringBuilder sb = new StringBuilder(); 15 if (Details != null && Details.Length > 0) 16 { 17 for (var i = 0; i < Details.Length; i++) 18 { 19 Detail detail = Details[i]; 20 21 StringBuilder sbThumbnails = new StringBuilder(); 22 string[] thumbnails = detail.Thumbnails; 23 if (thumbnails != null && thumbnails.Length > 0) 24 { 25 foreach (var thumbnail in thumbnails) 26 { 27 sbThumbnails.Append(thumbnail + ";"); 28 } 29 } 30 31 sb.AppendLine(String.Format("rnDetail{0}rnappKey={1}, cost={2}, createTime={3}, databagId={4}, fileId={5}, length={6}, name={7}, " 32 + "offlineDatabagStatus={8}, priority={9}, reason={10}, retry={11}, shareToken={12}, shareUrl={13}, sourceId={14}, status={15}, supportOfflineDatabag={16}, " 33 + "type={17}, thumbnails={18}", 34 (i + 1), detail.AppKey, detail.Cost, detail.CreateTime, detail.DatabagId, detail.FileId, detail.Length, detail.Name, 35 detail.OfflineDatabagStatus, detail.Priority, detail.Reason, detail.Retry, detail.ShareToken, detail.ShareUrl, detail.SourceId, detail.Status, detail.SupportOfflineDatabag, 36 detail.Type, sbThumbnails)); 37 } 38 } 39 40 sb.AppendLine("rnpage"); 41 sb.AppendLine(string.Format("prePage={0}, nextPage={1}, pageNo={2}, pageSize={3}, startIndex={4}, totalCount={5}, totalPages={6}", 42 Page.PrePage, Page.NextPage, Page.PageNo, Page.PageSize, Page.StartIndex, Page.TotalCount, Page.TotalPages)); 43 44 return string.Format("FileTranslateDetailsEntity [rn{0}rn]", sb); 45 } 46 }
1 /// <summary> 2 /// 轉換狀態詳情 3 /// </summary> 4 [Serializable] 5 public class Detail 6 { 7 /// <summary> 8 /// 應用的 appkey 9 /// </summary> 10 [JsonProperty("appKey")] 11 public string AppKey { get; set; } 12 13 /// <summary> 14 /// 任務耗時(單位:秒) 15 /// </summary> 16 [JsonProperty("cost")] 17 public int Cost { get; set; } 18 19 /// <summary> 20 /// 創建時間 21 /// </summary> 22 [JsonProperty("createTime")] 23 public string CreateTime { get; set; } 24 25 /// <summary> 26 /// 數據包id。例如:70b8c10b686061525420fc240bf48aca 27 /// </summary> 28 [JsonProperty("databagId")] 29 public string DatabagId { get; set; } 30 31 /// <summary> 32 /// 模型的fileId。例如:1609858191716512 33 /// </summary> 34 [JsonProperty("fileId")] 35 public long? FileId { get; set; } 36 37 /// <summary> 38 /// 文件長度(單位:位元組) 39 /// </summary> 40 [JsonProperty("length")] 41 public long? Length { get; set; } 42 43 /// <summary> 44 /// 集成模型的名稱,例如:integrate-test 45 /// </summary> 46 [JsonProperty("name")] 47 public string Name { get; set; } 48 49 /// <summary> 50 /// 離線數據包生成狀態:prepare(未生成) processing(生成中) success(生成成功) failed(生成失敗) 51 /// </summary> 52 [JsonProperty("offlineDatabagStatus")] 53 public string OfflineDatabagStatus { get; set; } 54 55 /// <summary> 56 /// 任務優先順序。 數字越大,優先順序越低。1, 2, 3。 57 /// </summary> 58 [JsonProperty("priority")] 59 public short? Priority { get; set; } 60 61 /// <summary> 62 /// 若轉換失敗,返回失敗原因。轉換成功時,返回空字元串 63 /// </summary> 64 [JsonProperty("reason")] 65 public string Reason { get; set; } 66 67 /// <summary> 68 /// 重試,true(或者false) 69 /// </summary> 70 [JsonProperty("retry")] 71 public bool? Retry { get; set; } 72 73 /// <summary> 74 /// 分享碼。例如:3c476c55 75 /// </summary> 76 [JsonProperty("shareToken")] 77 public string ShareToken { get; set; } 78 79 /// <summary> 80 /// 分享鏈接。例如:https://api.bimface.com/preview/3c476c55 81 /// </summary> 82 [JsonProperty("shareUrl")] 83 public string ShareUrl { get; set; } 84 85 /// <summary> 86 /// 模型對應的sourceId。該欄位暫時空置 87 /// </summary> 88 [JsonProperty("sourceId")] 89 public string SourceId { get; set; } 90 91 /// <summary> 92 /// 模型狀態,processing(處理中) success(成功) failed(失敗) 93 /// </summary> 94 [JsonProperty("status")] 95 public string Status { get; set; } 96 97 /// <summary> 98 /// 是否支援離線數據包,true(或者false) 99 /// </summary> 100 [JsonProperty("supportOfflineDatabag")] 101 public bool? SupportOfflineDatabag { get; set; } 102 103 /// <summary> 104 /// 模型的縮略圖,該欄位暫時空置 105 /// </summary> 106 [JsonProperty("thumbnail")] 107 public string[] Thumbnails { get; set; } 108 109 /// <summary> 110 /// 轉換類型。例如:rvt-translate(或者igms-translate…) 111 /// </summary> 112 [JsonProperty("type")] 113 public string Type { get; set; } 114 }
1 /// <summary> 2 /// 返回總記錄的分頁資訊 3 /// </summary> 4 [Serializable] 5 public class Page 6 { 7 /// <summary> 8 /// 下一頁碼 9 /// </summary> 10 [JsonProperty("nextPage")] 11 public int NextPage { get; set; } 12 13 /// <summary> 14 /// 當前頁碼 15 /// </summary> 16 [JsonProperty("pageNo")] 17 public int PageNo { get; set; } 18 19 /// <summary> 20 /// 每頁條目數 21 /// </summary> 22 [JsonProperty("pageSize")] 23 public int PageSize { get; set; } 24 25 /// <summary> 26 /// 上一頁碼 27 /// </summary> 28 [JsonProperty("prePage")] 29 public int PrePage { get; set; } 30 31 /// <summary> 32 /// 起始索引數 33 /// </summary> 34 [JsonProperty("startIndex")] 35 public int StartIndex { get; set; } 36 37 /// <summary> 38 /// 條目總數 39 /// </summary> 40 [JsonProperty("totalCount")] 41 public int TotalCount { get; set; } 42 43 /// <summary> 44 /// 頁碼總數 45 /// </summary> 46 [JsonProperty("totalPages")] 47 public int TotalPages { get; set; } 48 }