三、函數的參數
- 形參與實參(從函數調用的角度)
- 形式參數(parameter),簡稱形參,函數定義過程中小括弧裡面的參數;
- 實際參數(argument),簡稱實參, 指函數被調用過程中實際傳遞進來的參數。
>>> def my_function(param1, param2): # param1, param2為形參
return param1** 2 + param2 ** 2
>>> my_function(3, 4) # 3,4為實參
- 參數的具體類型
函數的參數具體可以有以下幾種分類:
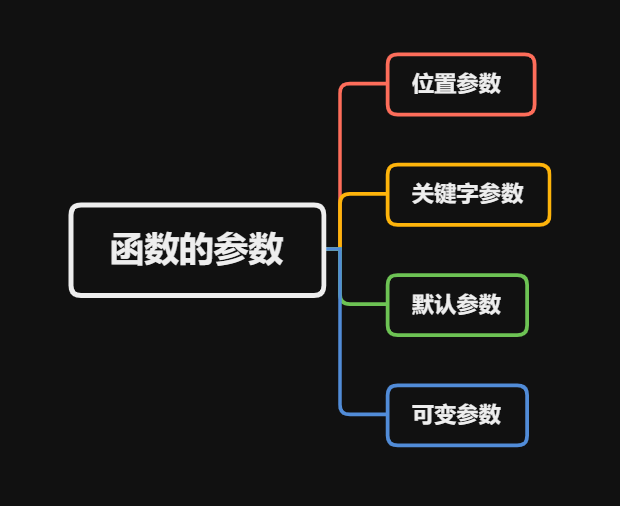
-
位置參數
位置固定的參數, 前面定義函數的參數都是位置參數 -
關鍵字參數
位置參數一多容易混淆, 因此在函數調用的時候引入這種映射類型的關鍵字參數就非常有用了
>>> def learn(teacher, student, course):
print(teacher + "教" + student + "學" + course)
>>> learn("王老師", "小明", "數學")
王老師教小明學數學
>>> learn("小明", "王老師", "數學")
小明教王老師學數學
>>> learn(student = "小明", course = "數學", teacher = "王老師")
王老師教小明學數學
>>> learn("王老師", student = "小明", course = "數學")
王老師教小明學數學
>>> learn(course = "數學", "小明", teacher = "王老師") # 位置參數必須在關鍵字參數的前面, 不然會出錯
SyntaxError: positional argument follows keyword argument
>>>
- 默認參數
python函數允許為參數指定默認值, 在函數調用的時候如果沒有傳入實參則採用默認值。
>>> def learn(teacher = "李老師", student = "小紅", course = "英語"):
print(teacher + "教" + student + "學" + course)
>>> learn()
李老師教小紅學英語
>>> learn("小明", "王老師", "數學")
小明教王老師學數學
>>> learn(student = "小明", course = "數學", teacher = "王老師")
王老師教小明學數學
>>> learn(student = "小明")
李老師教小明學英語
>>> def learn(teacher = "李老師", student = "小紅", course): # 在定義函數的時候如果有位置參數,則一定要在默認參數的前面,不然會報錯
print(teacher + "教" + student + "學" + course)
SyntaxError: non-default argument follows default argument
- 可變參數
之所以用可變參數(又叫收集參數),是因為函數的調用者有時也不知道要傳入多少個實參。
最典型的就是我們非常熟悉的print()函數
>>> print(1,2 ,3)
1 2 3
>>> print(1,2,3,4,5)
1 2 3 4 5
print()函數的定義就是採用了可變參數:
print(*objects, sep=’ ‘, end=’\n’, file=sys.stdout, flush=False)
Print objects to the text stream file, separated by sep and followed by end. sep, end, file and flush, if present, must be given as keyword arguments. python3.8官方文檔
可變參數在語法上有兩種方式:* 或者 **
>>> def output_alpha(*letter):
for i in letter:
print(i)
>>> output_alpha('a', 'b', 'c')
a
b
c
加(*)的可變參數實際上是把所有參數打包成一個元組。
>>> def output_alpha(*letter):
print(type(letter))
>>> output_alpha('a', 'b')
<class 'tuple'>
注意如果可變參數後面還需要指定其他參數,那麼在調用時(函數定義時沒啥要求,位置參數、默認參數都可)就應該使用關鍵字參數,不然python會把全部參數都納入到可變參數當中。
在函數的參數設置上,print()函數簡直就是我們的典範,再次把它掏出來:
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
如果要設置可變參數,那麼在傳入實參的時候重點肯定在可變參數上,因此不妨將其他參數都設置成默認參數,這樣簡直不要太方便靈活了。
另一種可變參數形式是用(**),不同之處時將所有參數打包成字典。
>>> def student(**info):
print(info)
>>> student(Amy=1, Tom=2, Bob=3)
{'Amy': 1, 'Tom': 2, 'Bob': 3}