深度學習中常用的影像數據增強方法-純乾貨
- 2019 年 11 月 13 日
- 筆記
微信公眾號:OpenCV學堂
影像數據增強方法概述
影像數據準備對神經網路與卷積神經網路模型訓練有重要影響,當樣本空間不夠或者樣本數量不足的時候會嚴重影響訓練或者導致訓練出來的模型泛化程度不夠,識別率與準確率不高!本文將會帶你學會如何對已有的影像數據進行數據增強,獲取樣本的多樣性與數據的多樣性從而為訓練模型打下良好基礎。通讀全文你將get到如何幾個技能:
- 使用標準化對影像進行影像增強
- 使用幾何變換(平移、翻轉、旋轉)對影像進行數據增強
- 使用隨機調整亮度對影像進行增強
- 使用隨機調整對比度對影像進行增強
演示基於mnist數據集,使用tensorflow+opencv,隨機獲取9張28×28的大小的數據影像,然後進行處理,處理之後通過opencv來顯示結果。載入mnisnt數據集,獲取隨機9張影像,顯示的程式碼如下:
from tensorflow.examples.tutorials.mnist import input_data import tensorflow as tf import numpy as np import cv2 as cv mnist = input_data.read_data_sets("MNIST_data/", one_hot=True) batch_xs, batch_ys = mnist.train.next_batch(9) def show_images(images_data, win_name): plot_image = np.zeros(shape=[96, 96], dtype=np.float32) for i in range(0, 9): col = i % 3 row = i // 3 plot_image[row*28:row*28+28, col*28:col*28+28] = images_data[i].reshape(28, 28) # show the plot cv.imshow(win_name, cv.resize(plot_image, (256, 256))) batch_xs = batch_xs.reshape(batch_xs.shape[0], 1, 28, 28) show_images(batch_xs, "batches") sess = tf.Session() print(batch_xs.shape)
選擇9張mnist影像

影像標準化
關於影像標準化的原理,可以看本公眾號以前的文章即可,點擊如下鏈接即可查看:
標準化的影像增強程式碼如下:
def standardization(): results = np.copy(batch_xs) for i in range(9): image = sess.run(tf.image.per_image_standardization(batch_xs[i].reshape(28, 28, -1))) results[i, :, :, :] = image.reshape(-1, 28,28) show_images(results, "standardization")
標準化增強如下

翻轉、旋轉
影像幾何變換通常包括影像的平移、翻轉、旋轉等操作,利用影像幾何操作實現影像數據增強。 翻轉操作程式碼如下:
def random_flip(): copy = np.copy(batch_xs) copy = np.squeeze(copy, axis=1) copy = np.expand_dims(copy, axis=3) flip_results = sess.run(tf.image.flip_left_right(copy)) flip_results = np.squeeze(flip_results, axis=3) flip_results = np.expand_dims(flip_results, axis=1) print(flip_results.shape) show_images(flip_results, "flip_left_right")
翻轉增強之後顯示

旋轉操作程式碼如下:
def random_rotate(): results = np.copy(batch_xs) for i in range(9): image = sess.run(tf.image.rot90(batch_xs[i].reshape(28, 28, -1), i%4+1)) results[i, :, :, :] = image.reshape(-1, 28,28) show_images(results, "random_rotate")
隨機90度旋轉操作增強之後
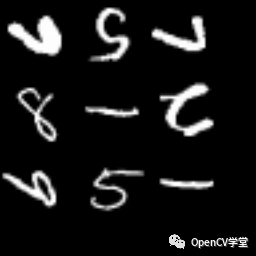
隨機亮度
隨機亮度通過調整影像像素值改變影像亮度,這種方式對影像進行數據增強的程式碼如下:
def random_brightness(): results = np.copy(batch_xs) for i in range(9): image = sess.run(tf.image.random_brightness(batch_xs[i].reshape(28, 28), 0.9)) results[i, :, :, :] = image.reshape(-1, 28,28) show_images(results,"random_brightness")
隨機亮度增強之後顯示

隨機對比度
隨機對比度,通過調整影像對比度來對影像進行數據增強,程式碼實現如下:
def random_contrast(): results = np.copy(batch_xs) for i in range(9): image = sess.run(tf.image.random_contrast(batch_xs[i].reshape(28, 28, -1), 0.85, 1.5)) results[i, :, :, :] = image.reshape(-1, 28,28) show_images(results, "random_contrast")
隨機對比度增強之後顯示

python運行調用
random_flip() random_brightness() random_contrast() random_rotate() standardization() cv.waitKey(0) cv.destroyAllWindows()